Apple Pay
A dependency free Cordova plugin to provide Apple Pay functionality.
https://github.com/samkelleher/cordova-plugin-applepay
Stuck on a Cordova issue?
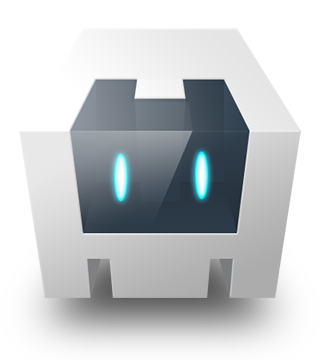
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation#
- Capacitor
- Cordova
- Enterprise
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
#
Supported Platforms- iOS
#
Usage#
ReactLearn more about using Ionic Native components in React